Stack pointer / Frame pointer 在 x86 Assembly 操作的簡易分析
這幾天在複習 compiler 有關 runtime environment 的章節,讀到 activation record 的時候發現對 frame pointer 的概念有點忘記了,於是就決定來做一點小實驗。
Toy example
|
|
x86 Assembly
把 assembly 編出來看一下 stack 操作的過程:
|
|
可以拿到:
|
|
把一些不重要的資訊精簡後,可以得到:
|
|
Analysis
Before foo
- 一開始在 main 被呼叫前,會在 glibc 的
_start
function 內,假設 SP 現在指到0x1924
FP 指在0x2000
- 接著保存 FP 的位址,將 FP 指到新的 SP 的位址
- 將 foo 要的參數 push 進 stack
- 呼叫 foo,保存 return address 在 stack
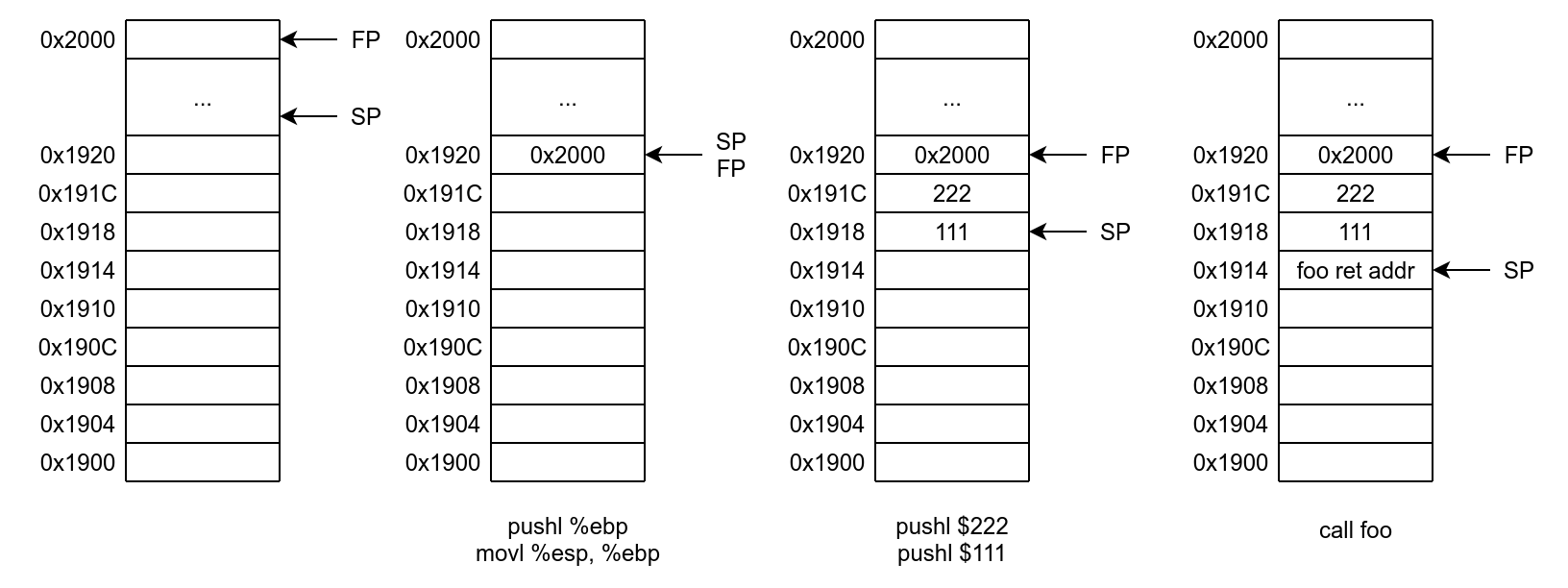
foo
- 進到 foo
- 保存 main 的 FP 的位址,將 FP 指到新的 SP 的位址
- SP - 16
- 算 x 與 y,透過 FP 拿到 local variables 的位址
- 將 return value 保存在
%eax
leave
將 SP 指回 FP,並 pop,FP 回到 main 原先呼叫的位址

After foo
- 回到 main
- SP 指向 FP 的位址
leave
將 SP 指回 FP,並 pop,FP 回到_start
原先呼叫的位址
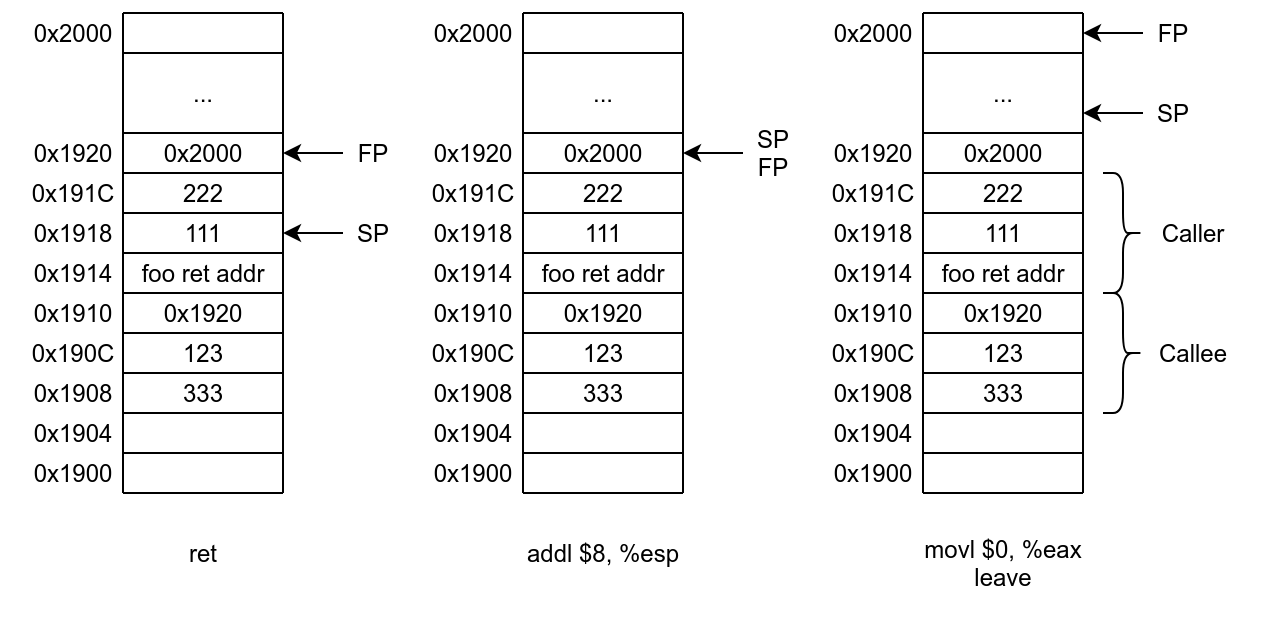